Value Range Event
A Value Range Event triggers events whenever the value of a Stat enters or leaves a certain threshold.
Add a Value Range Event
You can add Value Range Events with AddValueRangeEvent().
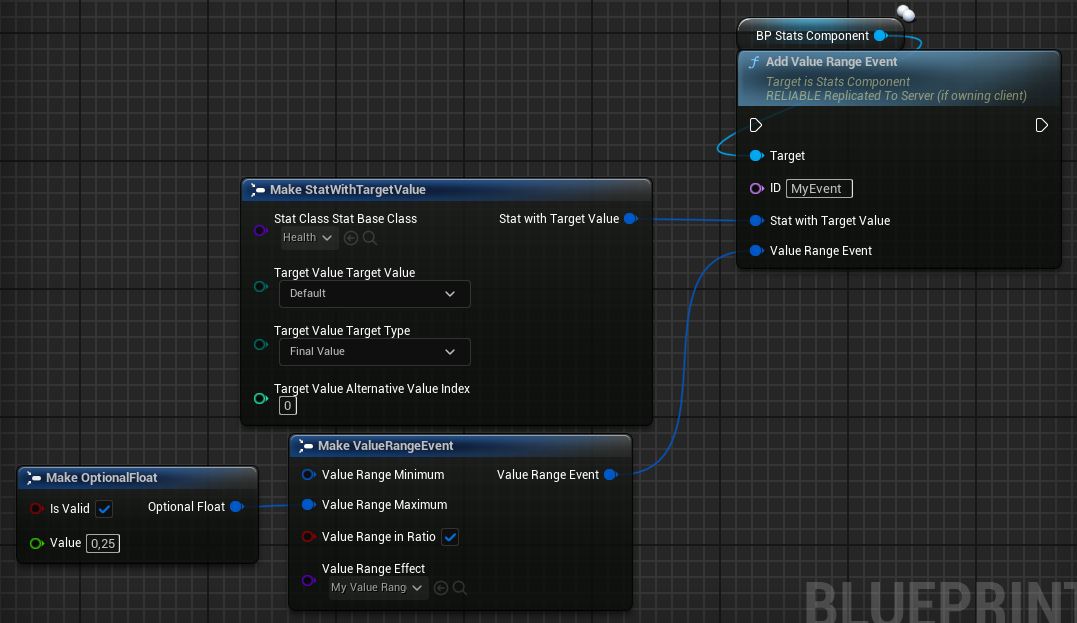
In this example, the EventTriggerIn() event of MyValueRangeEffect will be called when Health falls below 25%. EventTriggerOut() will be called when the Health value leaves the range.
Since the data is wrapped in structures, don't hesitate to break the structure pins to make it more readable.
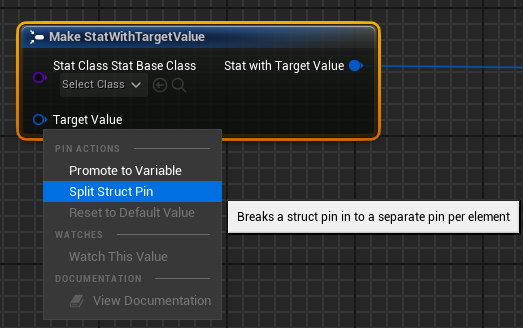
Stat With Target Value
This structure allows you to specify the target value that will trigger the events.
Here, I'm targeting the Final Value of Health.
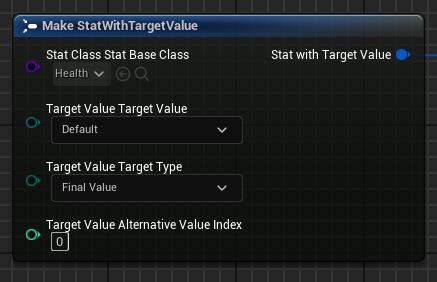
Here, I'm targeting the Alternative Value at index 1 of HealthMax.
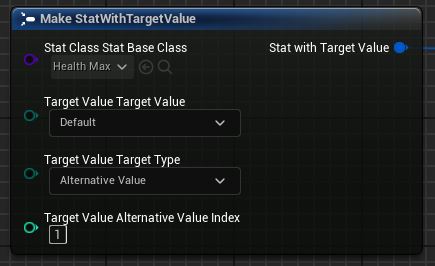
This structure is used in other areas of the code, which is why you have the Default / Minimum / Maximum / Regeneration Target Values.
For Value Range Events, since they only concern Stats, it is recommended to directly target the Stat you want to use by keeping the Target Value to
"Default".
Value Range
The Value Range structure is what determines the threshold.
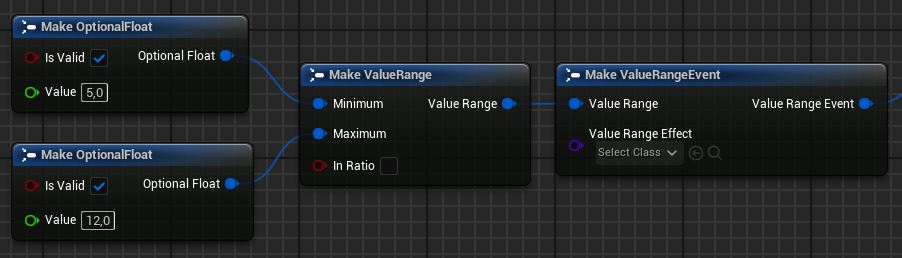
In this example, the Value Range Effect's EventTriggerIn() will be called when the value is between 5 and 12. EventTriggerOut() will be called when the value leaves that range.
You can also choose to only use Minimum or Maximum.

In this example, EventTriggerIn() will be called when the value is above 15. EventTriggerOut() will be called when the value is below 15.
Value Range Effect
The Value Range Effect contains the EventTriggerIn() and EventTriggerOut() functions that you can override.
Create a Value Range Effect
Create a Blueprint Class from the "Value Range Effect" class.
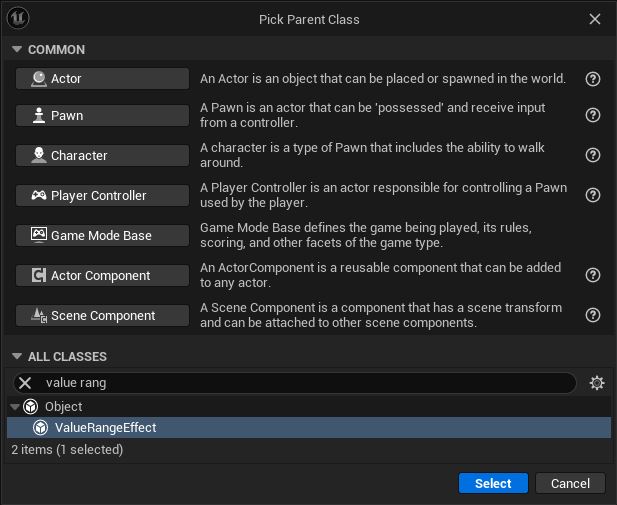
Open it and override either/both EventTriggerIn() and EventTriggerOut().
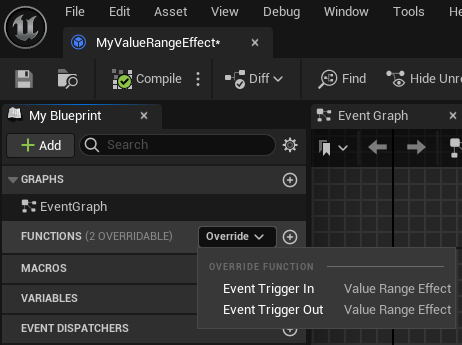
In any of those two functions, you'll have access to a bunch of informations to add your logic.
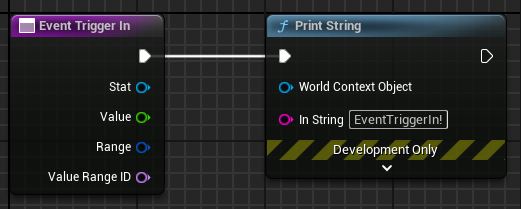
From here, you can get the StatsComponent by using Stat->GetOwnerStatsComponent().
Remove a Value Range Event
You can remove a Value Range Event with RemoveValueRangeEvent().
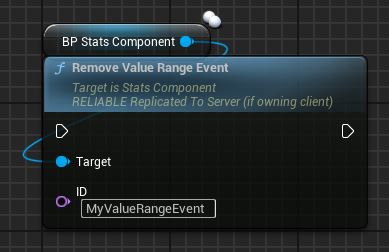
If you want to create a one-time effect, you can remove a Value Range Event directly from within EventTriggerIn() or EventTriggerOut().
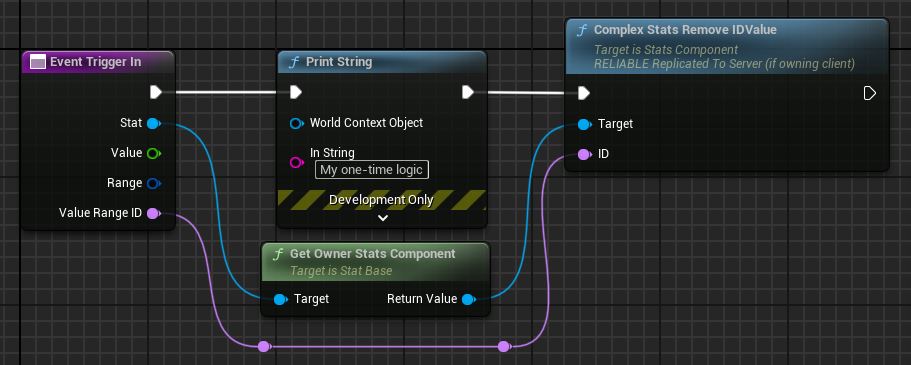
Example
Let's create a unique Perk "Cheat_Death": "The next time you take lethal damage, you regain 50% of your health. Single use.".
Whenever the Perk is added, I'll create a Value Range Event that triggers when my Health reaches 0%.
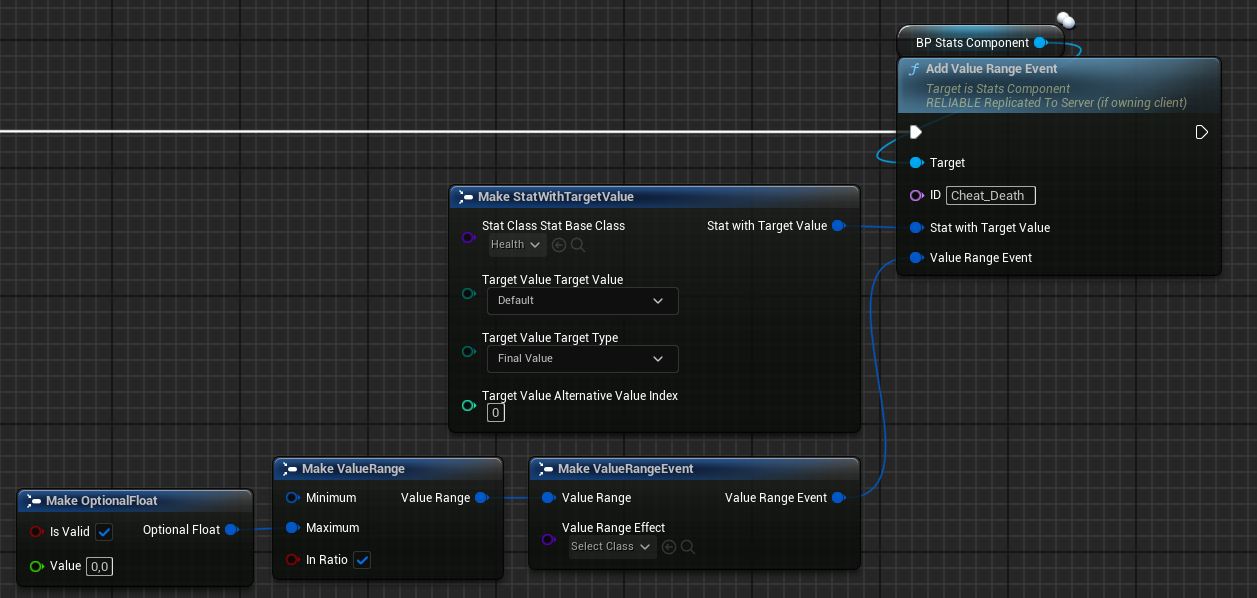
Now, I'll create a Value Range Effect "VRE_CheatDeath" and add it as the "Value Range Effect".
Override EventTriggerIn() and add the logic.
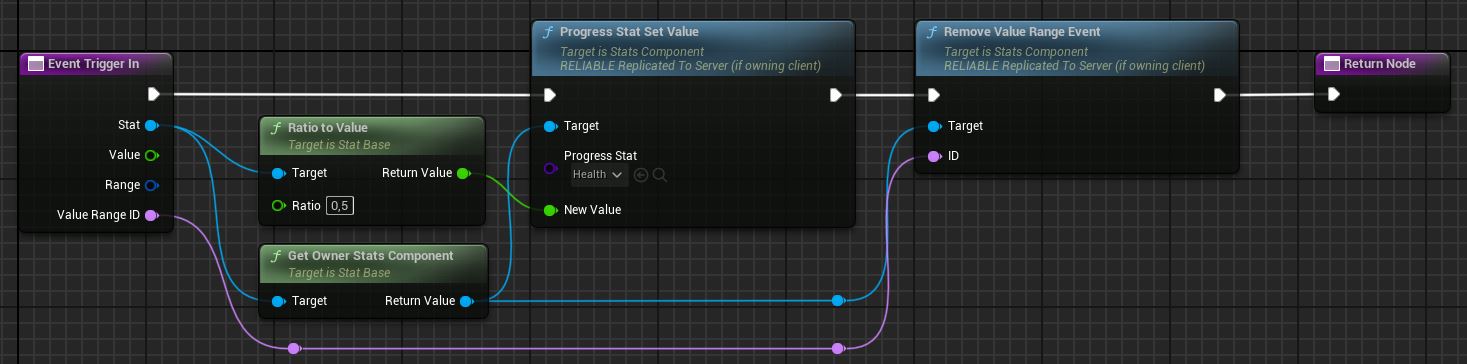
If you're using Value Range Events to trigger the death of your Character, both events might get called when Health reaches 0.
A solution is to remove the "Death" Value Range Event when you select the Perk, and add it back when EventTriggerIn()
is called.
When you add the Perk:
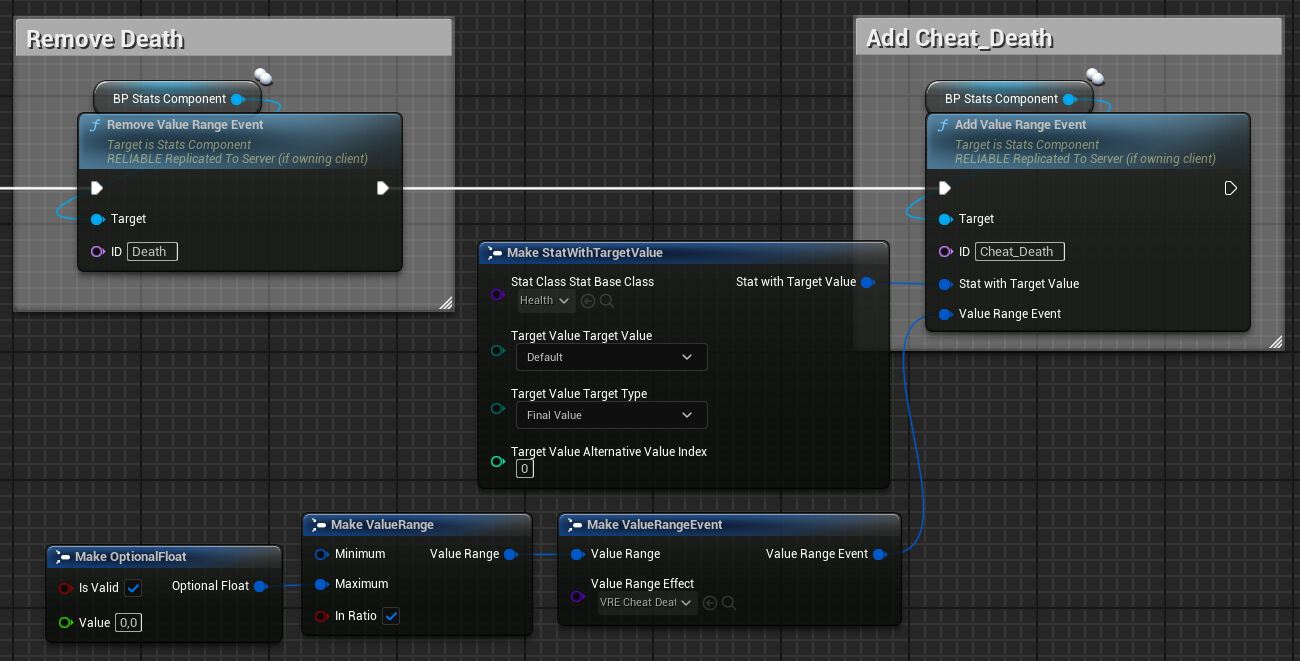
In VRE_CheatDeath:
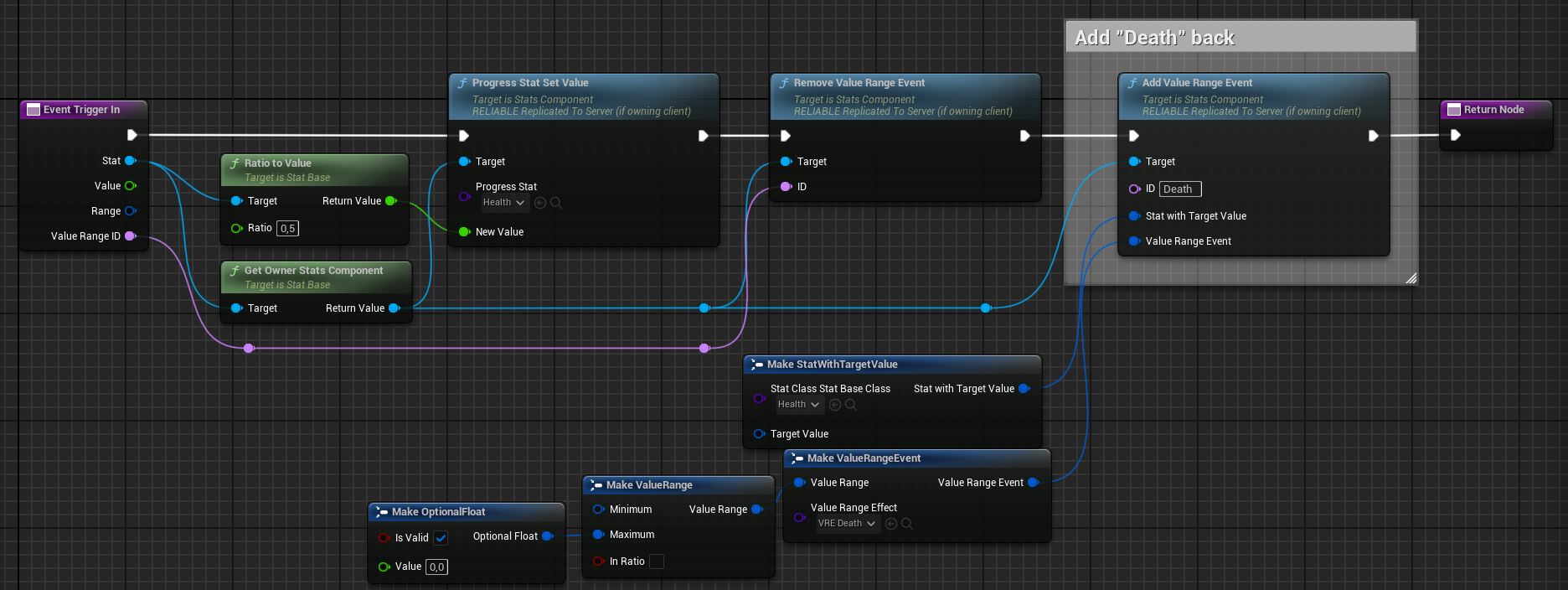
Using a Data Table
If you prefer, you can also put your Value Range Events in a Data Table, allowing you to only use their ID when applying them.
Create a Blueprint Structure
Create a Blueprint Structure with two variables: "Stat With Target Value" and "Value Range Event".
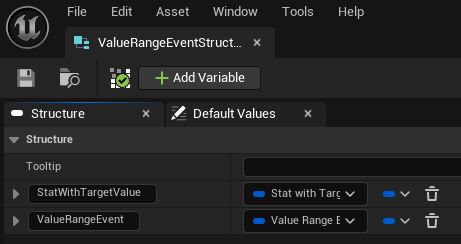
Create a Data Table with this structure
Create a Data Table using your new structure and add rows.
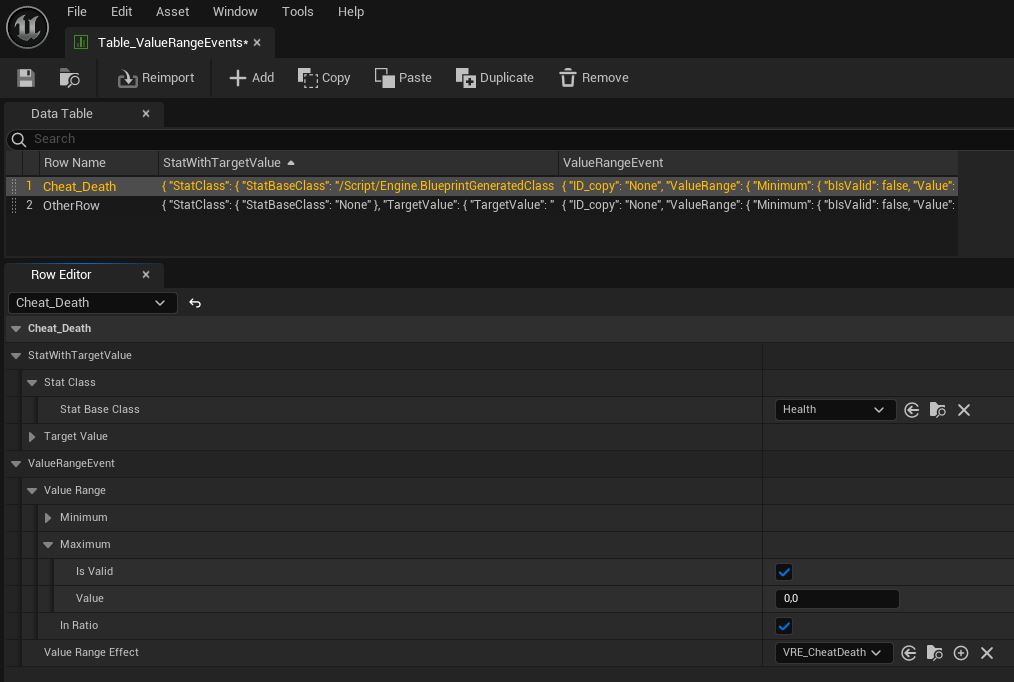
Use your Data Table
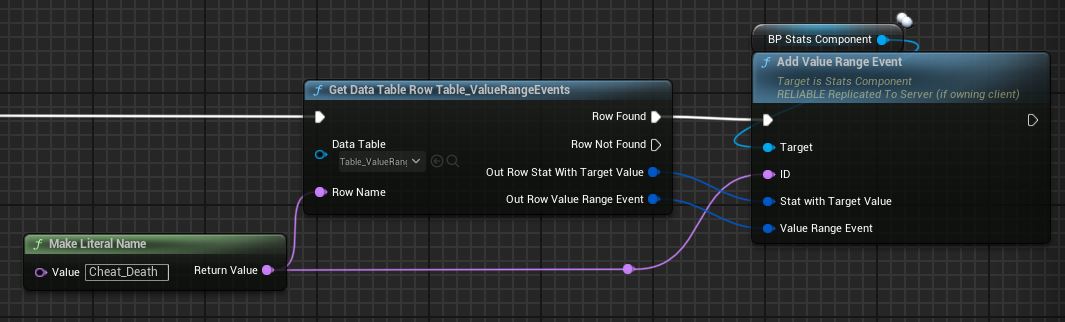